Changing a Related Join Field in Sequelize
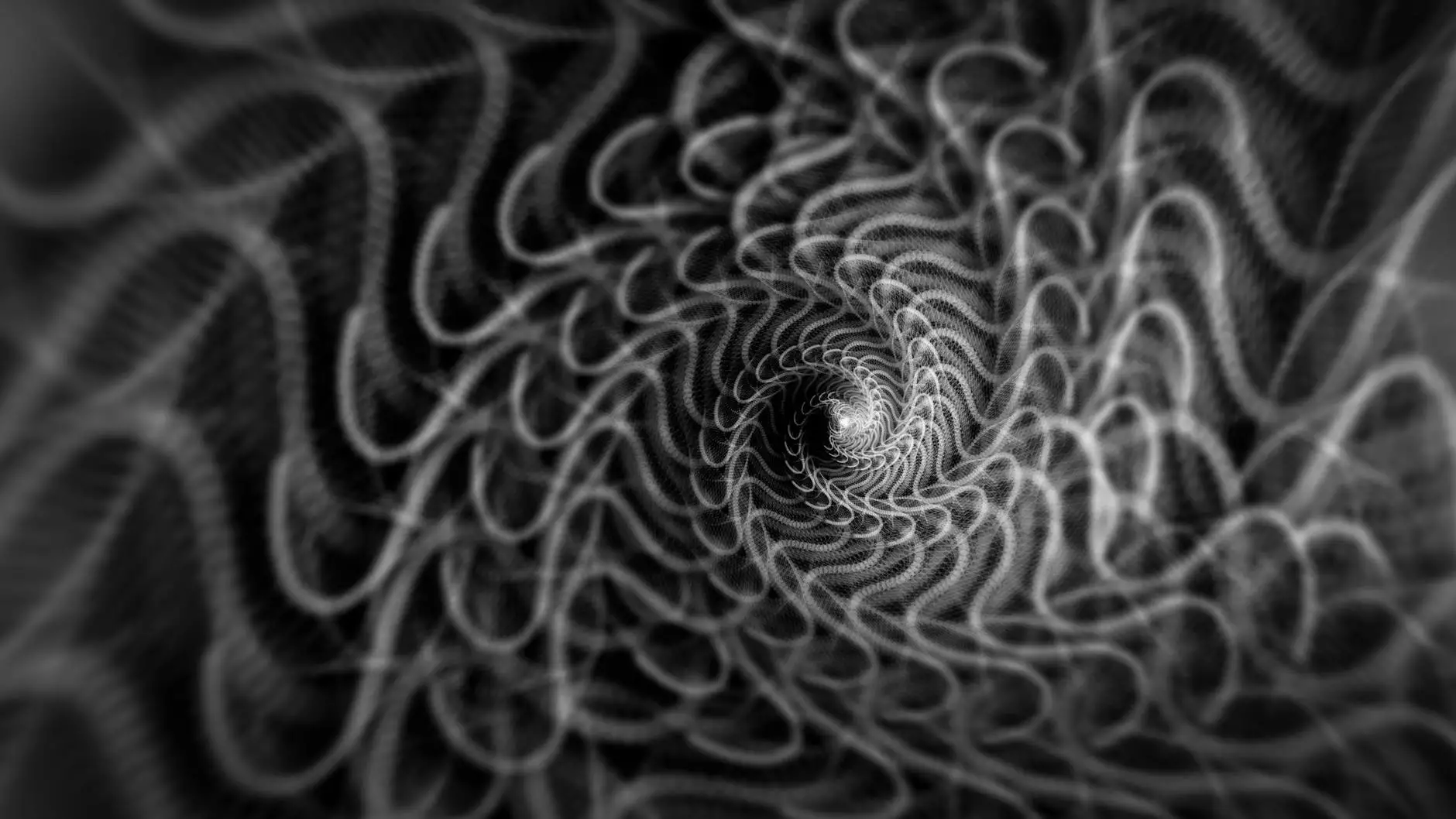
Introduction
When it comes to managing relationships between tables in a database, Sequelize is a popular Object-Relational Mapping (ORM) tool that offers a powerful and intuitive solution. In this guide, provided by SEO Martian, we will delve into the process of changing a related join field in Sequelize, enabling you to optimize and customize your database relationships with ease.
The Importance of Database Relationships
Database relationships play a critical role in ensuring efficient data management and retrieval. By establishing connections between tables, you can retrieve and combine related data effortlessly. Sequelize simplifies this process by providing an intuitive API that allows you to define and modify these relationships.
Understanding Sequelize Models
In Sequelize, models represent tables in your database. These models define the structure and behavior of your data, including the relationships between tables. Before we dive into changing related join fields, it's essential to have a solid understanding of Sequelize models.
Defining Models
To define a model in Sequelize, you need to create a JavaScript class that extends the Sequelize Model class. This class will serve as a blueprint for your corresponding database table. Within the model definition, you can specify attributes, associations, and various options to customize its behavior.
class User extends Model { static associate(models) { this.hasMany(models.Post, { foreignKey: 'userId', as: 'posts' }); } } User.init({ id: { type: DataTypes.INTEGER, primaryKey: true, autoIncrement: true, }, name: { type: DataTypes.STRING, allowNull: false, }, }, { sequelize, modelName: 'User', });Working with Associations
Associations define the relationships between models. In Sequelize, associations are handled through methods such as hasOne, hasMany, belongsTo, and belongsToMany. These methods allow you to establish relationships between tables using foreign keys and aliases.
Changing a Related Join Field
Now that we have a solid understanding of Sequelize models and relationships, let's explore how to change a related join field in Sequelize.
Scenario
Assume you have two models, User and Post, where each post belongs to a user. The current join field linking these models is userId. However, for specific reasons, you need to change this join field to authorId. This scenario requires a careful approach to ensure data integrity and maintain a smooth transition.
Step 1: Preparation
Prior to modifying the join field, it's crucial to take the following preparatory steps:
- Back up your database to safeguard against potential data loss.
- Identify all parts of your codebase that reference the existing join field userId.
- Update your codebase to reference the new join field authorId.
- Ensure that your codebase reflects any changes in the foreign key configuration.
Step 2: Migration
Next, create a Sequelize migration to modify the join field. Migrations are scripts that allow you to make changes to your database schema in a controlled and repeatable manner.
module.exports = { up: async (queryInterface, Sequelize) => { await queryInterface.renameColumn('Posts', 'userId', 'authorId'); }, down: async (queryInterface, Sequelize) => { await queryInterface.renameColumn('Posts', 'authorId', 'userId'); }, };By using the renameColumn method from the queryInterface object, we can modify the join field in a safe and controlled manner.
Step 3: Validation and Testing
Once the migration is created, it's essential to thoroughly test and validate the changes. Running test cases and validating the data integrity will help identify any potential issues early on and ensure a seamless transition.
Step 4: Executing the Migration
Finally, execute the migration to apply the changes to your database.
npx sequelize-cli db:migrateUpon successful execution, the join field linking the User and Post models will be updated to authorId, fulfilling your specific requirements.
Conclusion
In this comprehensive guide provided by SEO Martian, we have explored the process of changing a related join field in Sequelize. By following the outlined steps and best practices, you can seamlessly modify your database relationships while ensuring data integrity and optimal performance. Sequelize's flexibility and powerful features make it an excellent choice for managing complex database relationships.
For further assistance or inquiries regarding Sequelize or any other SEO services, please contact SEO Martian. We are here to help you achieve your database management and optimization goals.