iOS Swift Closures Quick Reference
About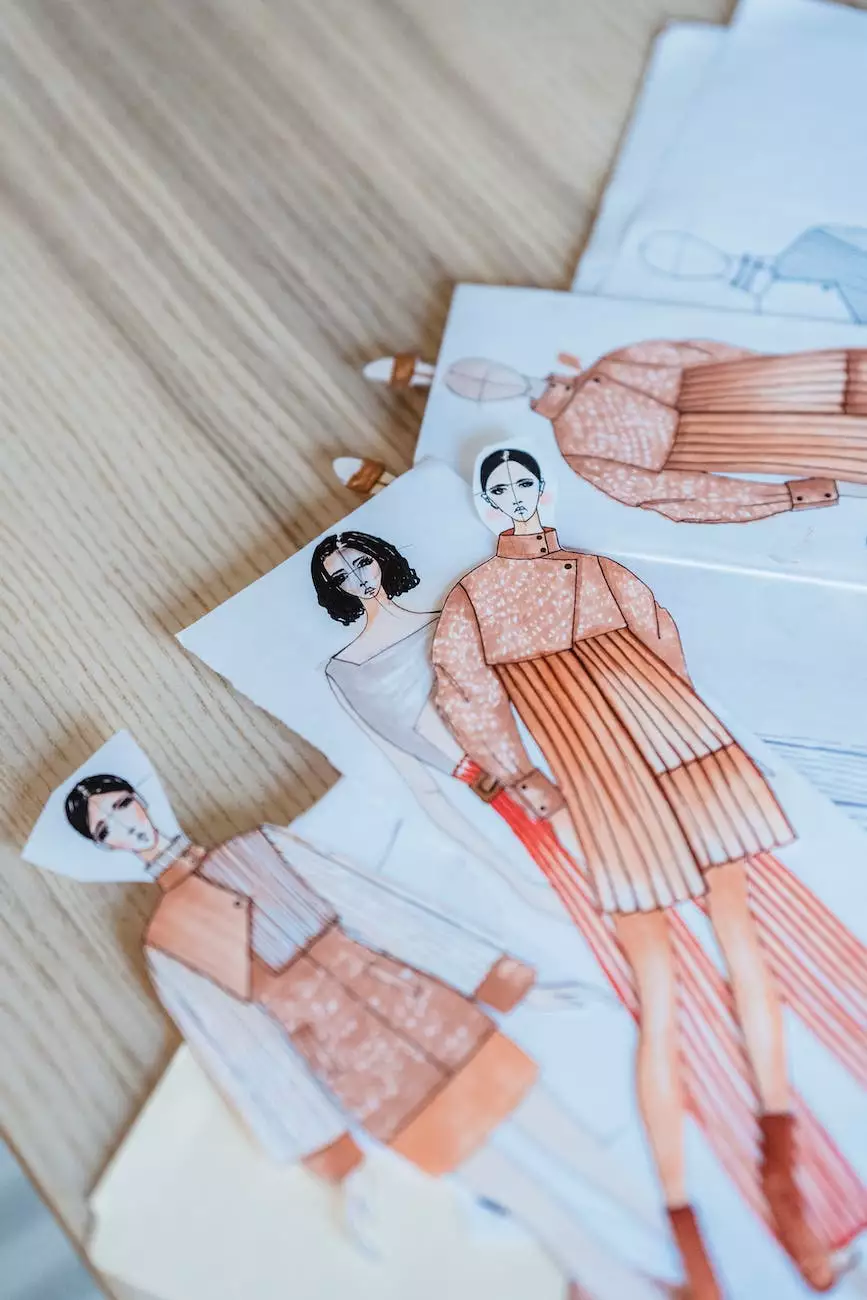
Understanding Swift Closures for iOS Development
Swift closures are powerful blocks of code that can be assigned to variables, passed as arguments, and stored within collections. As an iOS developer, having a solid understanding of closures is essential for writing efficient and flexible code.
The Syntax and Structure of Swift Closures
In Swift, closures are defined within {} brackets and can have separate parameters and a return type. They can capture and store references to variables and constants from the surrounding context, making them useful for encapsulating functionality.
Creating and Assigning Closures
To create a closure, you can use the following syntax:
let closureName: (parameters) -> ReturnType = { // Closure body }Here, closureName is the variable that holds the closure. The (parameters) defines the input parameters, and ReturnType represents the type of value the closure returns.
Using Closures as Arguments and Return Types
Closures can be passed as arguments to functions or methods and can also be returned as values. This allows for dynamic behavior and enables code reuse. A common use case is the map function:
let numbers = [1, 2, 3, 4, 5] let doubled = numbers.map({ (number: Int) -> Int in return number * 2 })Here, the closure is used as an argument to the map function, transforming each element of the numbers array by doubling it. The resulting array doubled will contain [2, 4, 6, 8, 10].
Key Concepts in Swift Closures
Capture Lists
Closures can capture and store references to variables and constants. By default, Swift captures these values by reference, meaning they can be modified within the closure. However, when capturing value types like structs, it's important to use capture lists to avoid capturing a reference to the entire instance.
Trailing Closures
Trailing closures allow you to move the closure outside of the function parentheses, improving code readability. They are particularly useful when the closure is the last argument passed to a function. Here's an example:
func performAction(completion: () -> Void) { // Perform some action completion() } performAction() { print("Action completed.") }Here, the closure { print("Action completed.") } is passed as a trailing closure to the performAction function.
Escaping Closures
Escaping closures are closures that outlive the function they are passed into. They must be marked with the @escaping attribute to indicate that they can be stored and called outside of the original function's execution scope. This is commonly used when dealing with asynchronous operations or completion handlers.
Conclusion
Swift closures are a powerful tool for iOS developers to write flexible and reusable code. By understanding their syntax, structure, and key concepts, you can leverage closures to enhance the functionality and efficiency of your iOS apps. Dive deeper into the world of Swift closures and unlock new possibilities for your iOS development journey.
SEO Martian - Your Partner in Business and Consumer Services
SEO Martian provides top-notch SEO services to businesses in various industries. With our expertise in search engine optimization, we help your website outrank the competition and reach the top spots on Google search results.
Why Choose SEO Martian?
- Proven track record of delivering exceptional SEO results
- Experienced team of SEO specialists who stay updated with the latest industry trends
- Customized strategies tailored to your business goals and target audience
- Comprehensive website analysis and optimization to improve ranking and visibility
- Transparent reporting and regular performance monitoring
- Affordable pricing plans that suit businesses of all sizes
- Excellent customer support and dedicated account managers
Our SEO Services
- Keyword research and analysis
- On-page optimization
- Off-page optimization
- Technical SEO audit
- Content creation and optimization
- Link building
- Local SEO
- Mobile SEO
- Ecommerce SEO
- SEO consulting and strategy development
Contact SEO Martian Today
Take your online presence to new heights with SEO Martian. Contact us today to discuss your SEO needs and get started on the path to dominating the search engine rankings!