Understanding React Context API
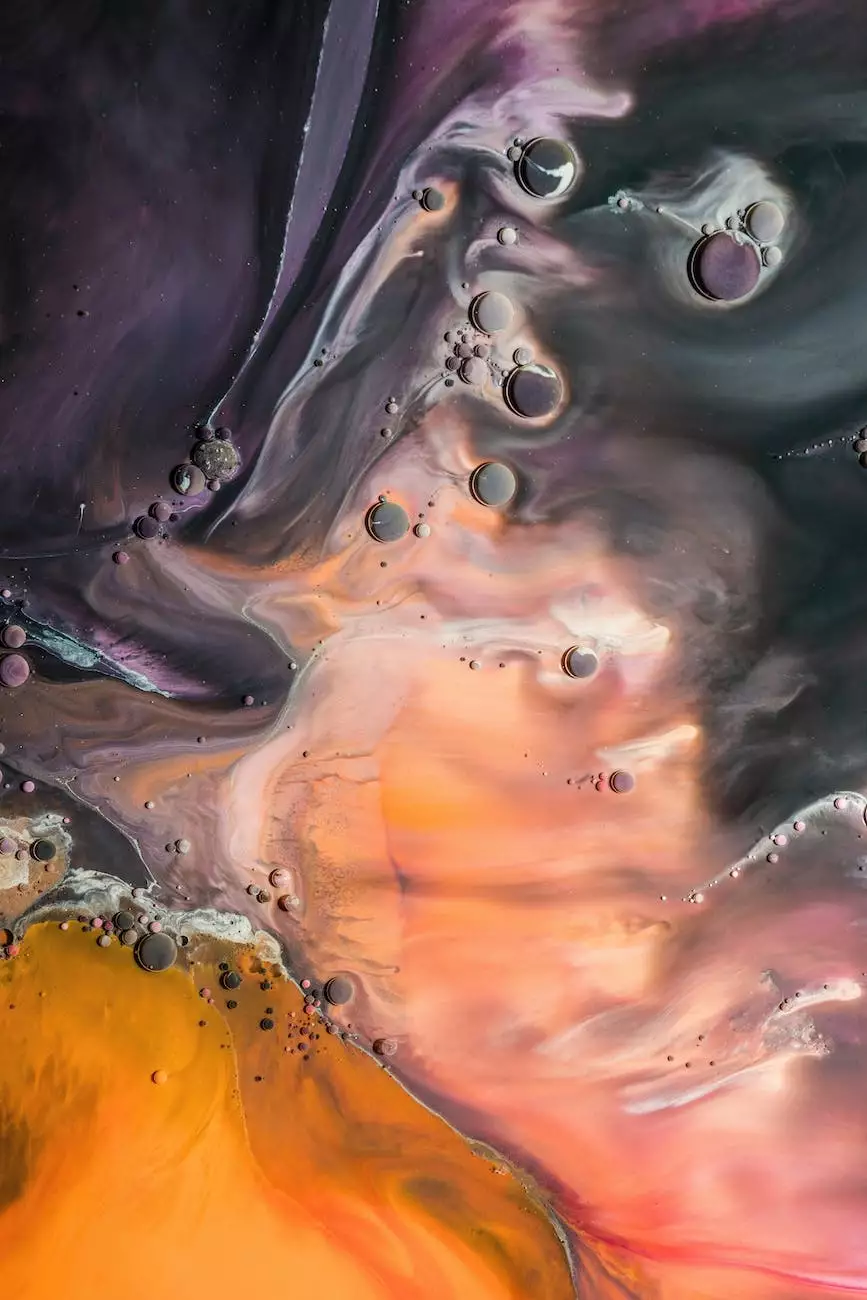
Introduction
Welcome to SEO Martian, the leading provider of SEO services in the Business and Consumer Services category. In this article, we will dive deep into the details of the React Context API. With our comprehensive guide, you will gain a solid understanding of how to leverage this powerful feature in your web development projects.
What is the React Context API?
The React Context API is a feature of React, a popular JavaScript library for building user interfaces. It allows you to share data across various components without passing props through intermediate components. This makes your code more maintainable, scalable, and reduces the need for prop drilling.
Benefits of Using React Context API
By utilizing the React Context API, you can streamline your development process and enhance the performance of your application. Some key benefits include:
- Simplified State Management: With React Context API, you can easily manage and update the application state, reducing the complexity of your codebase.
- Global Data Access: The Context API enables you to make data accessible to all components within the context, eliminating the need for manual prop passing.
- Enhanced Code Reusability: Contexts can be reused across different parts of your application, promoting code reusability and reducing redundant code.
- Efficient Performance: The React Context API is designed to optimize performance by only re-rendering the components that depend on the changed context value.
- Flexible Architecture: Context API provides flexibility in designing your application's architecture by allowing you to create multiple contexts for different sets of data.
How to Use React Context API
Step 1: Creating a Context
To start using the React Context API, you need to create a context using the createContext method from the react package.
import React from 'react'; const MyContext = React.createContext();Step 2: Providing and Consuming Context
After creating a context, you can provide it to the relevant components using the Provider component and consume the context using the Consumer component or utilizing the useContext hook.
import React from 'react'; const MyContext = React.createContext(); const MyComponent = () => { return ( {/* Your components */} {(contextValue) => { return ( {/* Consume the context value */} {contextValue} ); }} {/* Using useContext hook */} const contextValue = React.useContext(MyContext); ); };Step 3: Updating Context Value
You can update the context value using the Provider's value prop. When the context value changes, all the components consuming that particular context will be automatically updated and re-rendered.
import React, { useState } from 'react'; const MyComponent = () => { const [value, setValue] = useState('initial-value'); return ( setValue('new-value')}> Update Context Value {/* Other components */} ); };Best Practices
To ensure optimal utilization of the React Context API, consider the following best practices:
- Context Granularity: Avoid excessive nesting of context providers and maintain an appropriate granularity level for better code organization.
- Separation of Concerns: Separate unrelated contexts to keep your codebase organized and maintainable.
- Performance Optimization: Implement memoization techniques or use the React.memo() component to prevent unwanted re-renders when consuming contexts.
- Testing Considerations: Make use of context providers and mock the values provided by the context while testing your components.
- Error Handling: Implement a fallback strategy when the context value is undefined or unavailable.
Conclusion
In this comprehensive guide, we have explored the React Context API from its basics to advanced usage. By leveraging the power of the React Context API, you can enhance the state management and performance of your React applications. SEO Martian, the leading SEO service provider in the Business and Consumer Services category, is here to help you implement the React Context API effectively in your web development projects. Contact us today to take advantage of our SEO services and stay ahead of the competition.